Sending SMS messages from your WIX website can easily be done through Twilio if you have Corvid (Dev mode) enabled for your site. In this article I will explain how to do it.
Note: You’ll need an active Twilio account with a phone number for this to work.
Preparing Functionality
First of all, go to your node_modules
folder inside the Corvid editor and install twilio
. Wix already has the library available, so why not take advantage of it.
To keep your code organized, create a utils
folder inside your Backend
folder to store our helper file.
Create a new file called twilio.js
(the name is up to you), I just kept it simple.
You should see a your backend folder looking similar to this:
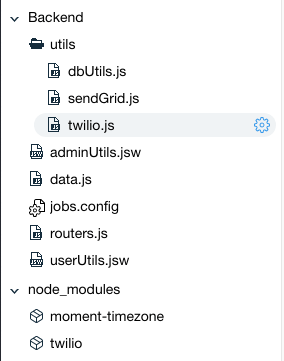
Now, open the new twilio.js
file and add this code:
// Function used to send text messages via Twilio
export function sendText(phone, body) {
const accountSid = "ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"; // Your Account SID from www.twilio.com/console
const authToken = "your_auth_token"; // Your Auth Token from www.twilio.com/console
const client = require("twilio")(accountSid, authToken); // ignore Wix error
client.messages
.create({
body: body, // Dynamic value from incoming parameters
from: "+12345678901", // Your Twilio number from www.twilio.com/console
to: phone, // Dynamic value from incoming parameters
})
.then((message) => console.log(message.sid));
}
Note: We created the helper function sendText
so that you could send SMS messages from any part of your code, without exposing account credentials. (Of course, in a regular Nodejs environment, you would load those credentials from environment variables anyway, instead of including them in the code.)
Sending Messages
To send messages, you will import the helper function wherever you want to use it.
Once imported in a file (backend files only), you can send a message by calling the sendText
function.
import { sendText } from "backend/utils/twilio";
const textDestination = "+19876543210"; // Phone number you wish to send to
const message = "Thank you for joining us!"; // Message text you want to send
// Send text message using Twilio helper function
sendText(textDestination, message)
.then((response) => {
console.log("Sent text: " + message);
})
.catch((error) => {
console.log("Error sending text: ", error);
});
That’s it!
Conclusion
This functionality can be expanded to many use cases (automatic SMS on form submissions, daily/weekly SMS updates, lead nurturing, etc.).
If you have any questions or would like me to write another article with a specific use case, let me know in the comments below!
The code from this article, along with other interesting snippets for WIX/Corvid can be found on GitHub.